- Home ›
- Categories ›
- Flutter
5 Things I do when starting a new Flutter project
No matter how small or large, there are 5 things I do every time when starting a new Flutter project.
1.) Thinking about a project name
Although it sounds trivial, the first thing I do when creating a new Flutter project is to think about how I want to name the project. This is necessary for naming the project directory.
The name of the project will also be part of the bundle identifier in iOS and the package name in Android. They can be a bit tiresome to change, so better think of a meaningful name right away.
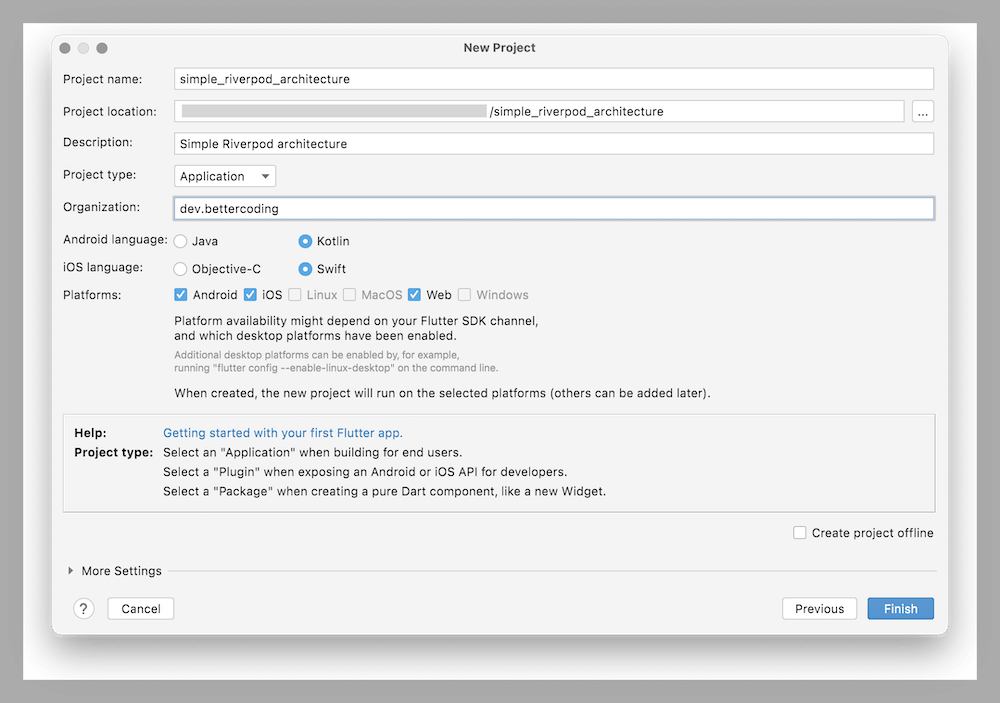
2.) Clean up pubspec.yaml
By default, pubspec.yaml
includes a tremendous number of comments. Although they might be useful if you are new to Flutter, I like to get rid of all of them. In my opinion, the comments make it hard to understand the content of the file.
3.) Clean up main.dart
Next, I like to remove all the sample code from the main.dart
file. Usually, this is what my main.dart
file looks like when I start a new project:
import 'package:flutter/material.dart';
import 'package:simple_riverpod_architecture/home_page.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
}
Code language: Dart (dart)
Additionally, I added a new file called home_page.dart
and added the most basic implementation:
import 'package:flutter/material.dart';
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const Scaffold(
body: Center(child: Text('Hello World')),
);
}
}
Code language: JavaScript (javascript)
4.) Run the app
Now, that I have the most basic app possible, I will run the project. This way, I make sure everything is set up successfully. Since Flutter supports hot reload, I now also see my develop process immediately as I code along.
5.) Initialize git
Lastly, I initialize version control on the project. This can be simply done by running
git init
git add .
git commit -m "initial commit"
Code language: JavaScript (javascript)
This initializes git on the projects, adds all files and creates a first commit. Having set up version control allows me to check what changes I have made since the last commit and lets me roll back changes.
I do not set up an origin right away, this I do later when I decide I want to have my project saved in a repository.
What about you?
Do you have other/additional steps that you do on every new Flutter project? Let me know in the comments below.
Leave a Reply